import matplotlib.pyplot as plt
import geopandas as gpd
import rasterio
import rasterio.plot
import contextily as cx
import folium
8 Making maps with Python
Prerequisites
This chapter requires importing the following packages:
It also relies on the following data files:
= gpd.read_file('data/nz.gpkg')
nz = gpd.read_file('data/nz_height.gpkg')
nz_height = rasterio.open('data/nz_elev.tif')
nz_elev = gpd.read_file('data/world.gpkg', where='name_long="Tanzania"')
tanzania = tanzania.to_crs(32736).buffer(50000).to_crs(4326)
tanzania_buf = gpd.read_file('data/world.gpkg', mask=tanzania_buf) tanzania_neigh
8.1 Introduction
A satisfying and important aspect of geographic research is communicating the results. Map making—the art of cartography—is an ancient skill that involves communication, intuition, and an element of creativity. In addition to being fun and creative, cartography also has important practical applications. A carefully crafted map can be the best way of communicating the results of your work, but poorly designed maps can leave a bad impression. Common design issues include poor placement, size and readability of text and careless selection of colors, as outlined in the style guide of the Journal of Maps. Furthermore, poor map making can hinder the communication of results (Brewer 2015):
Amateur-looking maps can undermine your audience’s ability to understand important information and weaken the presentation of a professional data investigation.
Maps have been used for several thousand years for a wide variety of purposes. Historic examples include maps of buildings and land ownership in the Old Babylonian dynasty more than 3000 years ago and Ptolemy’s world map in his masterpiece Geography nearly 2000 years ago (Talbert 2014).
Map making has historically been an activity undertaken only by, or on behalf of, the elite. This has changed with the emergence of open source mapping software such as mapping packages in Python, R, and other languages, and the “print composer” in QGIS, which enable anyone to make high-quality maps, enabling “citizen science”. Maps are also often the best way to present the findings of geocomputational research in a way that is accessible. Map making is therefore a critical part of geocomputation and its emphasis not only on describing, but also changing the world.
Basic static display of vector layers in Python is done with the .plot
method or the rasterio.plot.show
function, for vector layers and rasters, as we saw in Sections Section 1.2.2 and Section 1.3.1, respectively. Other, more advanced uses of these methods, were also encountered in subsequent chapters, when demonstrating the various outputs we got. In this chapter, we provide a comprehensive summary of the most useful workflows of these two methods for creating static maps (Section 8.2). Static maps can be easily shared and viewed (whether digitally or in print), however they can only convey as much information as a static image can. Interactive maps provide much more flexibilty in terms of user experience and amount of information, however they often require more work to design and effectively share. Thus, in Section 8.3, we move on to elaborate on the .explore
method for creating interactive maps, which was also briefly introduced earlier in Section 1.2.2.
8.2 Static maps
Static maps are the most common type of visual output from geocomputation. For example, we have been using .plot
and rasterio.plot.show
throughout the book, to display geopandas and rasterio geocomputation results, respectively. In this section we systematically review and elaborate on the various properties that can be customized when using those functions.
A static map is basically a digital image. When stored in a file, standard formats include .png
and .pdf
for graphical raster and vector outputs, respectively. Thanks to their simplicity, static maps can be shared in a wide variety of ways: in print, through files sent by e-mail, embedded in documents and web pages, etc.
Nevertheless, there are many aesthetic considerations when making a static map, and there is also a wide variety of ways to create static maps using novel presentation methods. This is the focus of the field of cartography, and beyond the scope of this book.
Let’s move on to the basics of static mapping with Python.
8.2.1 Minimal examples
A vector layer (GeoDataFrame
) or a geometry column (GeoSeries
) can be displayed using their .plot
method (Section 1.2.2). A minimal example of a vector layer map is obtained using .plot
with nothing but the defaults (Figure 8.1).
; nz.plot()
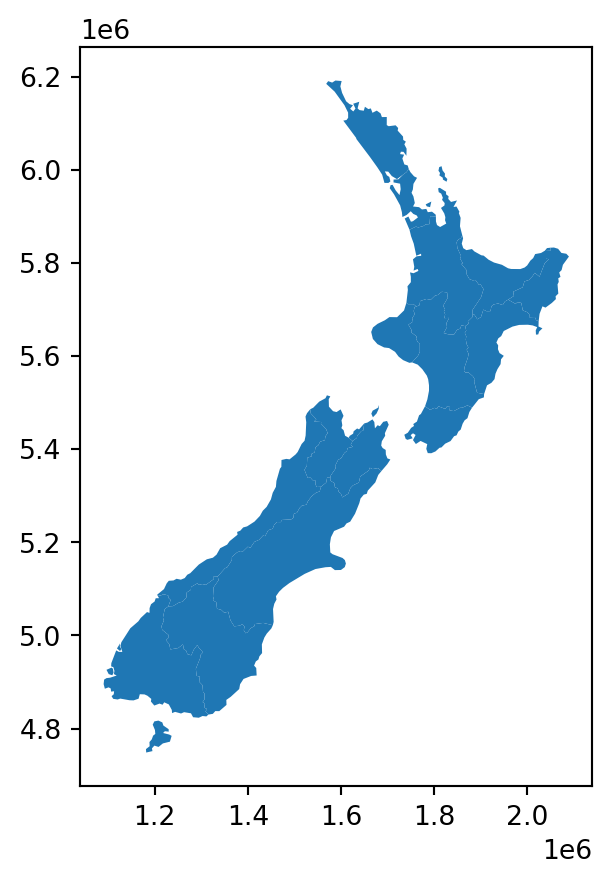
.plot
A rasterio
raster file connection, or a numpy ndarray
, can be displayed using rasterio.plot.show
(Section 1.3.1). Figure 8.2 shows a minimal example of a static raster map.
; rasterio.plot.show(nz_elev)
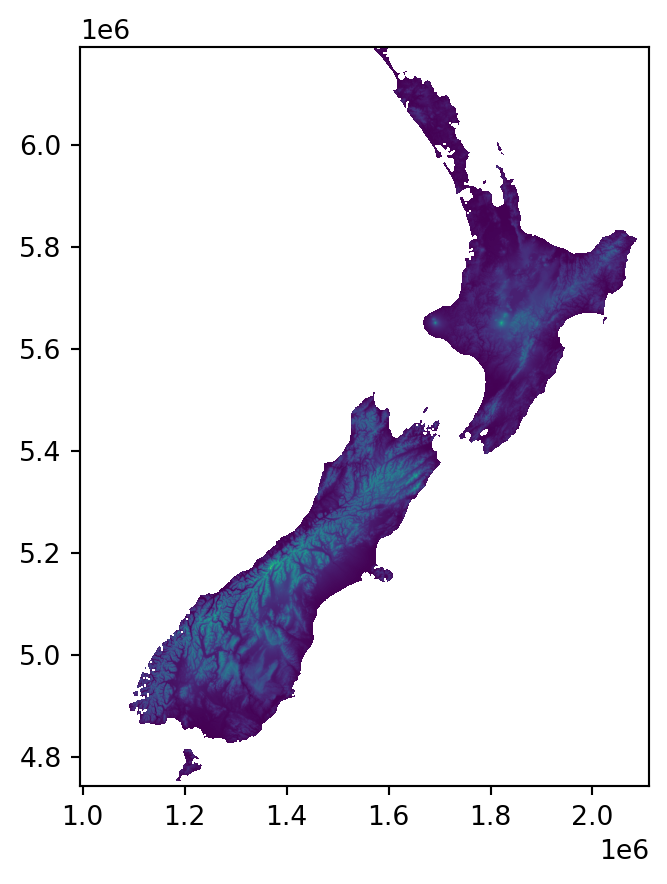
rasterio.plot.show
8.2.2 Styling
The most useful visual properties of the geometries, that can be specified in .plot
, include color
, edgecolor
, and markersize
(for points) (Figure 8.3).
='lightgrey');
nz.plot(color='none', edgecolor='blue');
nz.plot(color='lightgrey', edgecolor='blue'); nz.plot(color
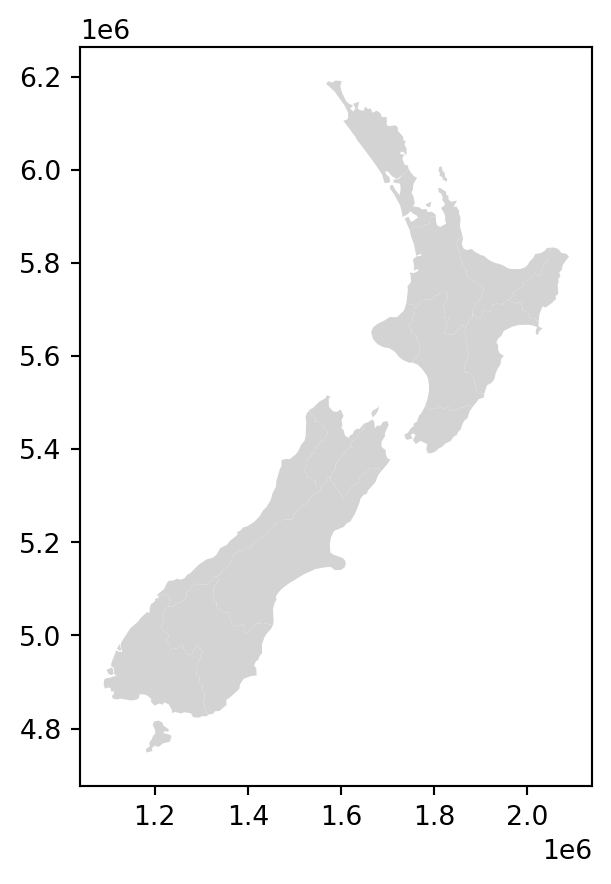
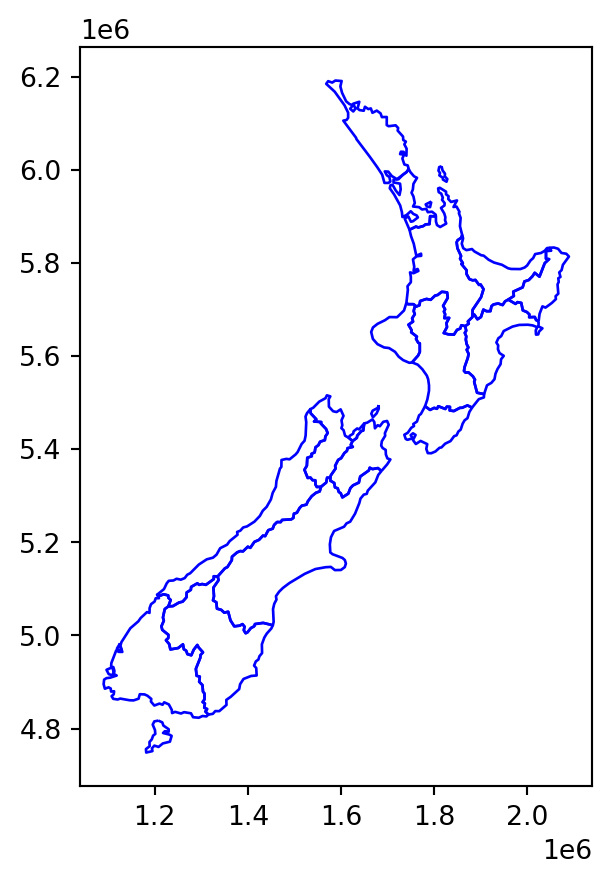
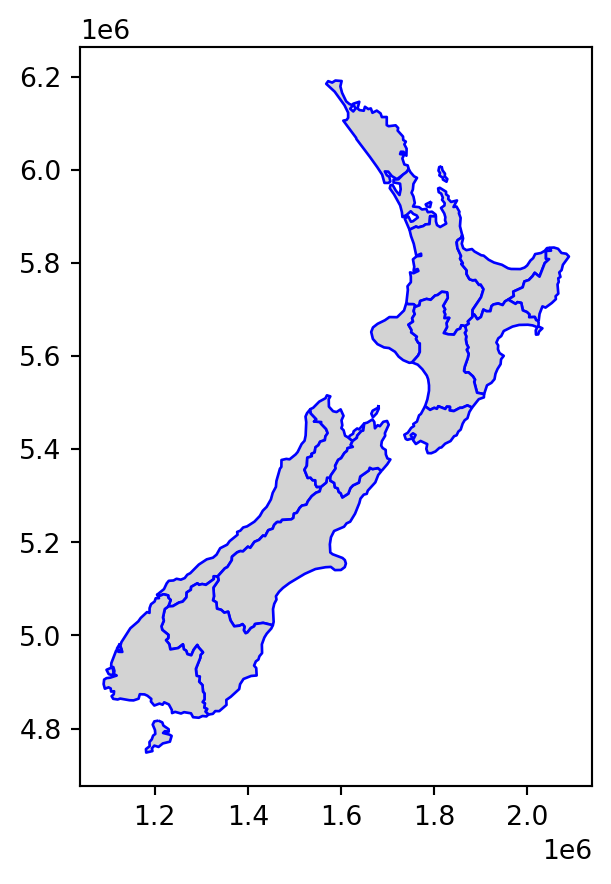
color
and edgecolor
in static maps of a vector layer
The next example uses markersize
to get larger points (Figure 8.4). It also demonstrates how to control the overall figure size, such as \(4 \times 4\) \(in\) in this case, using plt.subplots
to initialize the plot and its figsize
parameter to specify dimensions.
= plt.subplots(figsize=(4,4))
fig, ax =100, ax=ax); nz_height.plot(markersize
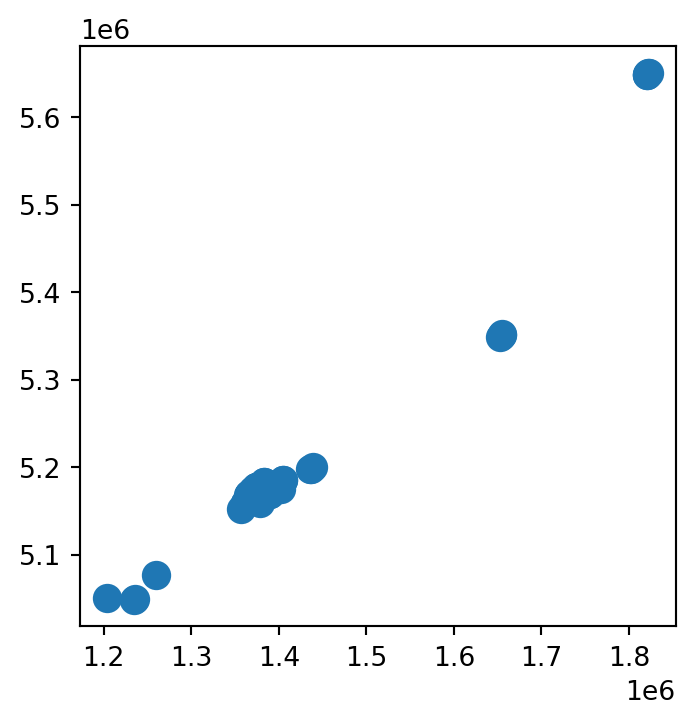
markersize
in a static map of a vector layer
As you have probably noticed throughout the book, the plt.subplots
function is used to initialize a maptplotlib plot layout, possibly also specifying image size (e.g., Figure 8.4) and multi-panel layout (e.g., Figure 8.17). The returned value is a tuple
of Figure
and Axes
objects, which we conventionally unpack to variables named fig
and ax
. These two variables represent the entire figure, and the elements of individual sub-figures, respectively.
For our purposes in this book, we have been using just the ax
object, passing it to the ax
parameter in further function calls, in order to add subsequent layers (e.g., Figure 8.15) or other elements (e.g., Figure 8.10) into the same panel. In a single-panel figure, we pass ax
itself, whereas in a multi-panel figure we pass individual elements representing a specific panel (such as ax[0]
or ax[0][0]
, depending of the layout; see Section 8.2.7)
Note that in some of the cases we have used an alternative to plt.subplots
—we assigned an initial plot into a variable, conventionally named base
, similarly passing it to the ax
parameter of further calls, e.g., to add subsequent layers (e.g., Figure 8.14); this (shorter) syntax, though, is less general than plt.subplots
and not applicable in some of the cases (such as displaying a raster and a vector layer in the same plot, e.g., Figure 8.15).
8.2.3 Symbology
We can set symbology in a .plot
using the following parameters:
column
—a column namelegend
—whether to show a legendcmap
—color map, a.k.a. color scale, a palette from which the colors are sampled
For example, Figure 8.5 shows the nz
polygons colored according to the 'Median_income'
attribute (column), with a legend.
='Median_income', legend=True); nz.plot(column
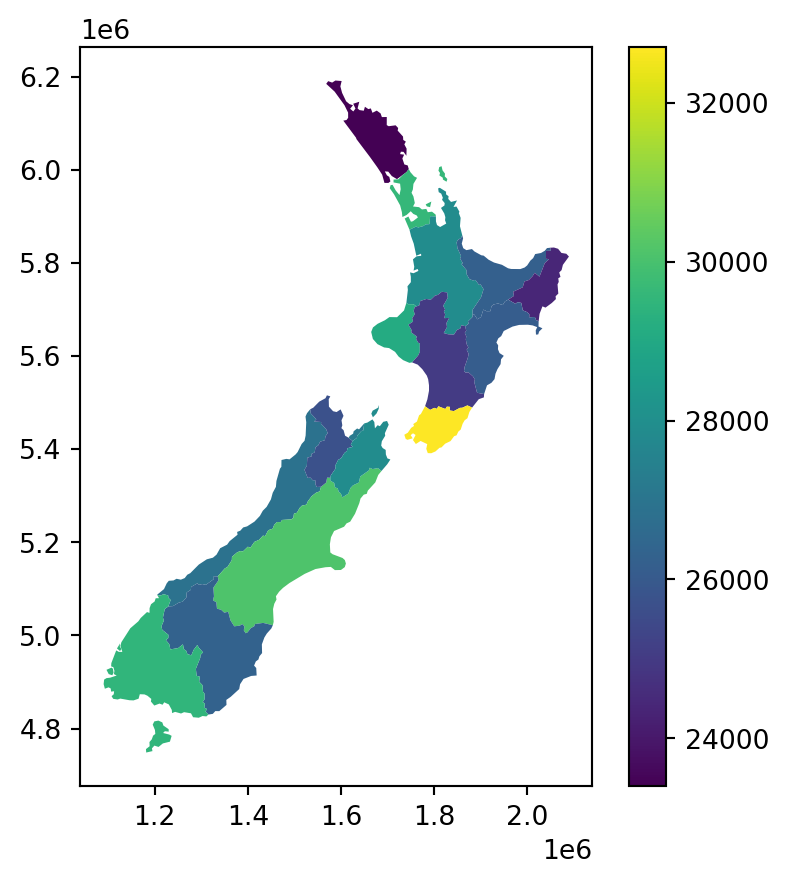
.plot
The default color scale which you see in Figure 8.5 is cmap='viridis'
. The cmap
(“color map”) argument can be used to specify one of countless color scales. A first safe choice is often the ColorBrewer collection of color scales, specifically designed for mapping. Any color scale can be reversed, using the _r
suffix. Finally, other color scales are available: see the matplotlib colormaps article for details. The following code sections demonstrates three color scale specifications other than the default (Figure 8.6).
='Median_income', legend=True, cmap='Reds');
nz.plot(column='Median_income', legend=True, cmap='Reds_r');
nz.plot(column='Median_income', legend=True, cmap='plasma'); nz.plot(column
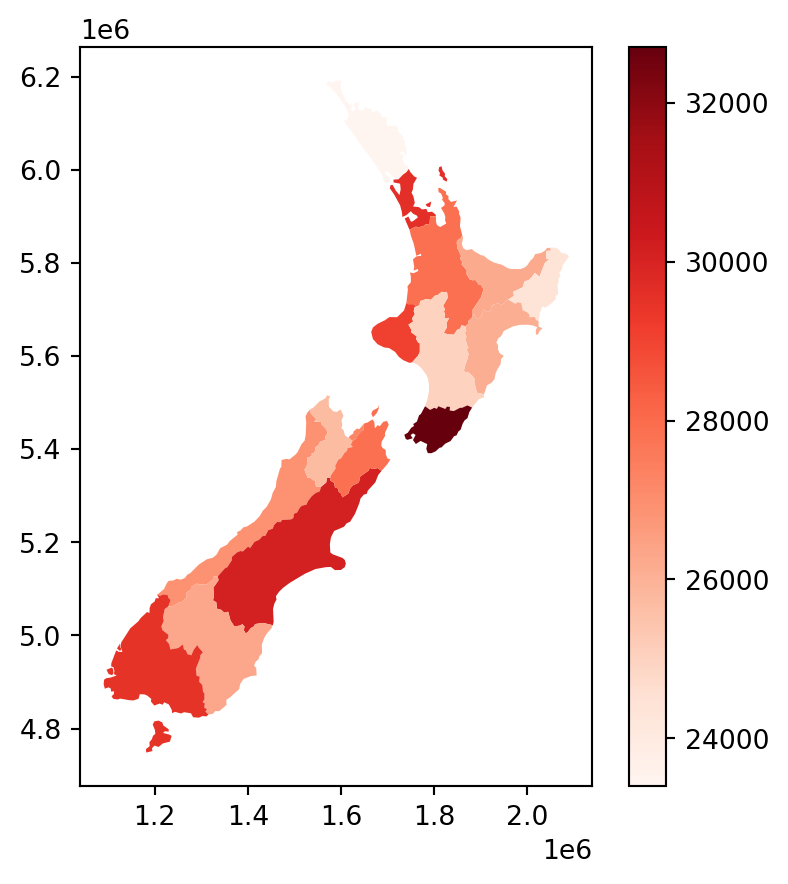
'Reds'
color scale from ColorBrewer
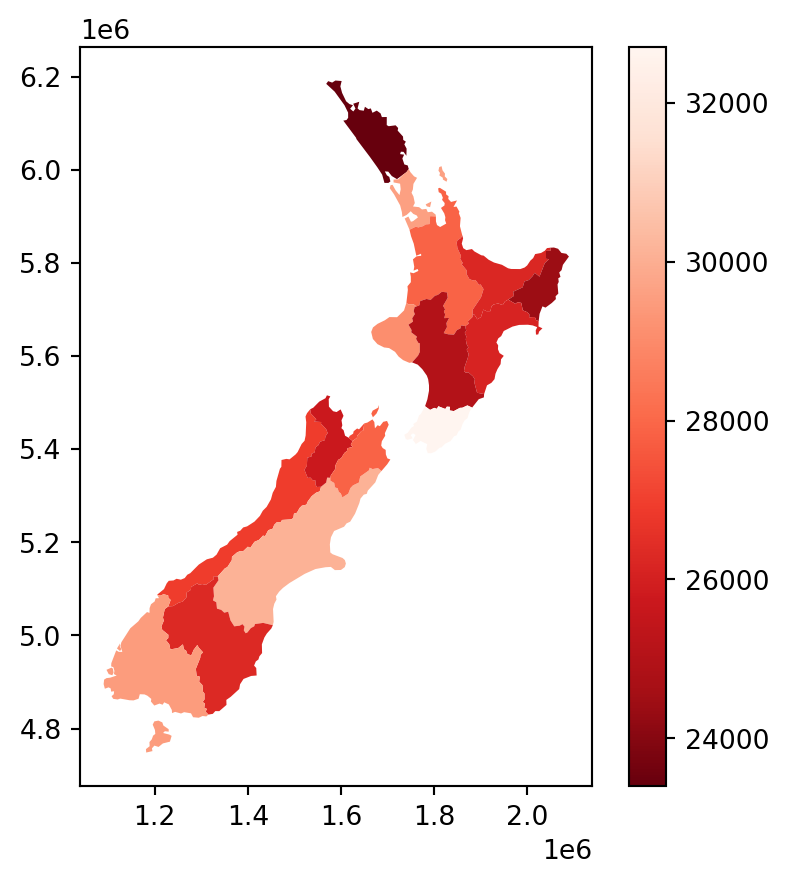
'Reds'
color scale
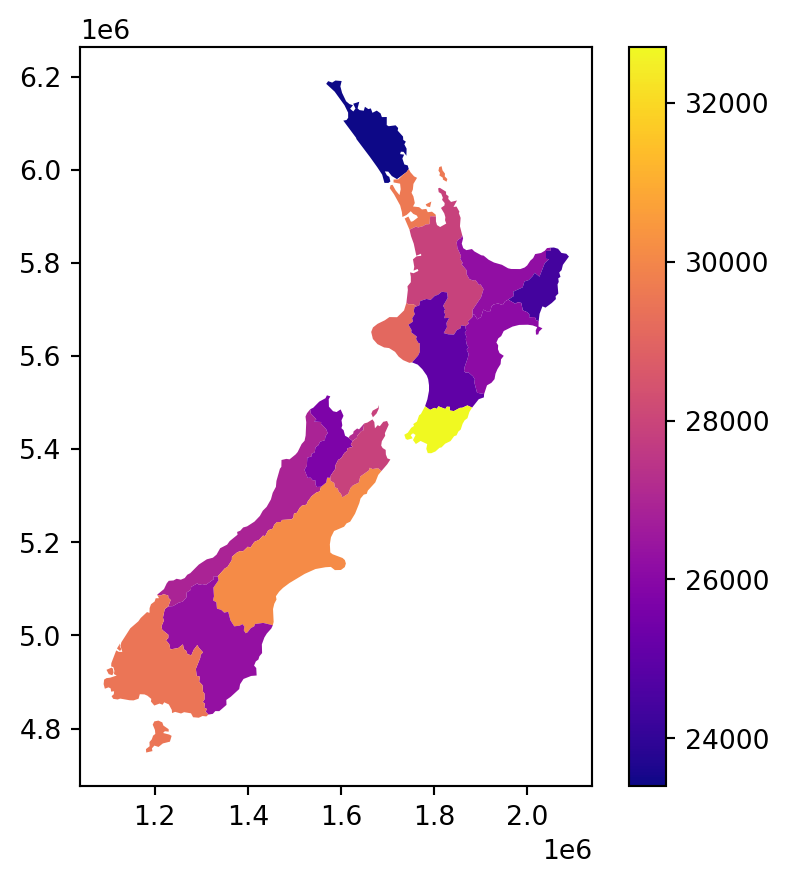
'plasma'
color scale from matplotlib
.plot
Categorical symbology is also supported, such as when column
points to an str
attribute. For categorical variables, it makes sense to use a qualitative color scale, such as 'Set1'
from ColorBrewer. For example, the following expression sets symbology according to the 'Island'
column (Figure 8.7).
='Island', legend=True, cmap='Set1'); nz.plot(column
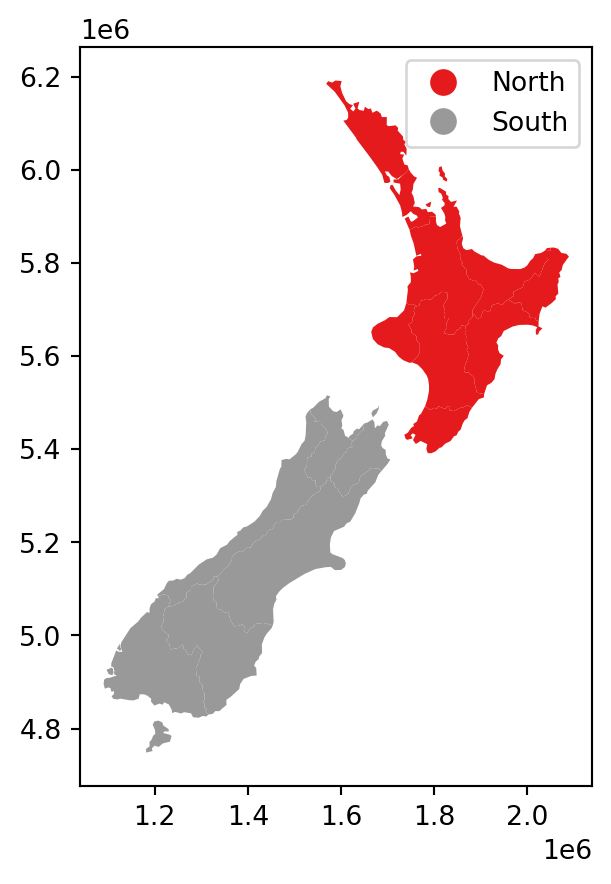
In case the legend interferes with the contents (such as in Figure 8.7), we can modify the legend position using the legend_kwds
argument (Figure 8.8).
='Island', legend=True, cmap='Set1', legend_kwds={'loc': 4}); nz.plot(column
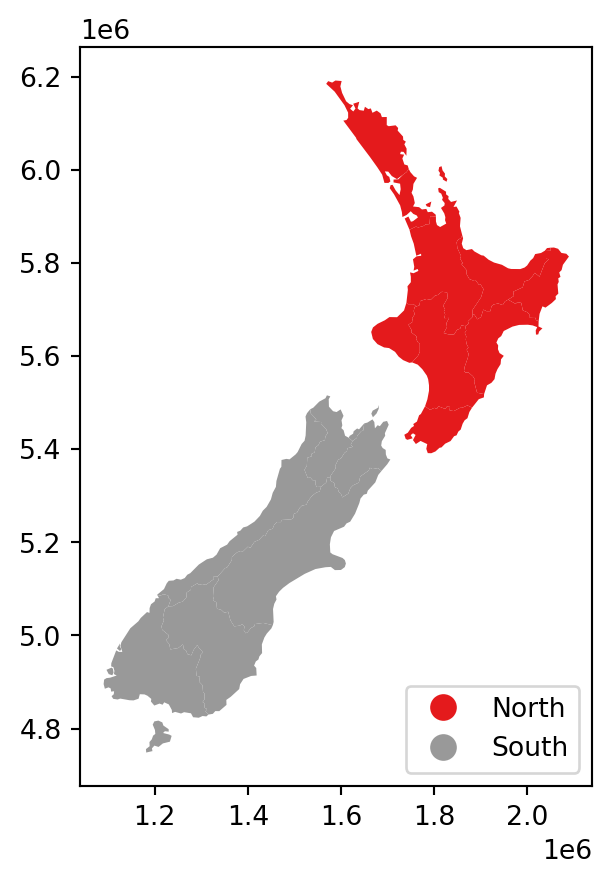
.plot
The rasterio.plot.show
function is also based on matplotlib (Hunter 2007), and thus supports the same kinds of cmap
arguments (Figure 8.9).
='BrBG');
rasterio.plot.show(nz_elev, cmap='BrBG_r');
rasterio.plot.show(nz_elev, cmap='gist_earth'); rasterio.plot.show(nz_elev, cmap
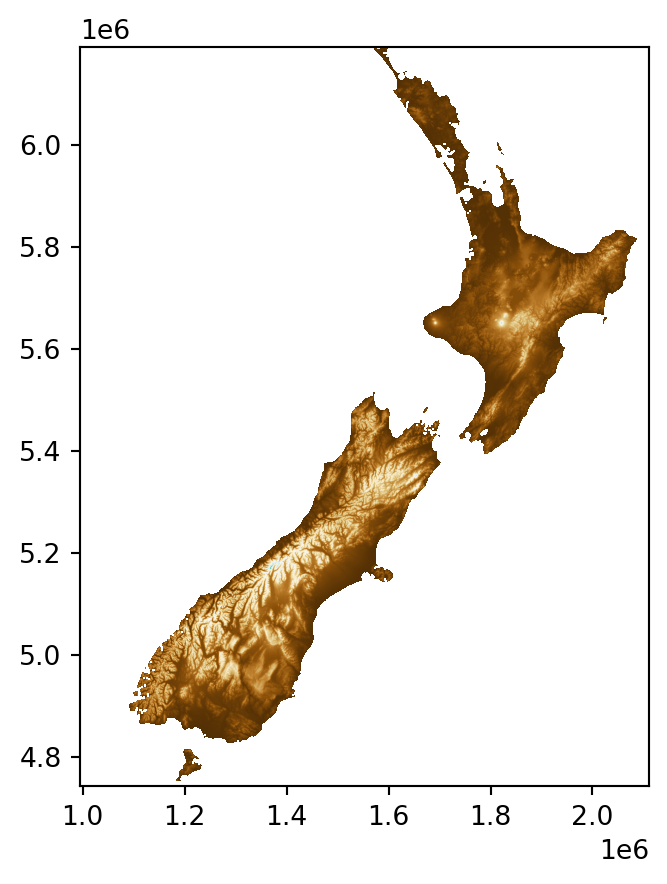
'BrBG'
color scale from ColorBrewer
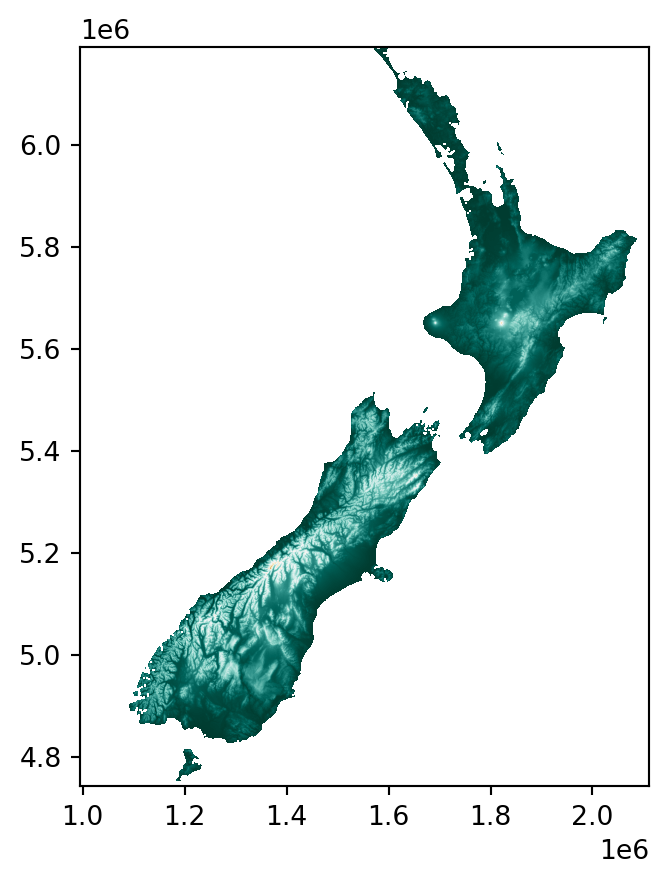
'BrBG_r'
color scale
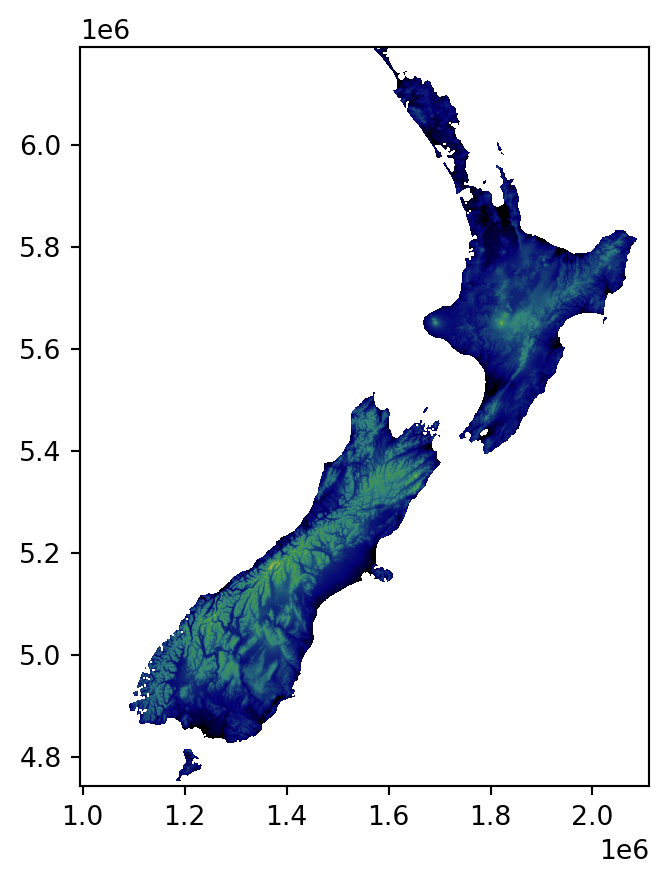
'gist_earth'
color scale from matplotlib
rasterio.plot.show
Unfortunately, there is no built-in option to display a legend in rasterio.plot.show
. The following workaround, reverting to matplotlib methods, can be used to acheive it instead (Figure 8.10). Basically, the code reverts to the matplotlib .colorbar
method to add a legend, using the plt.imshow
function that draws an image of a numpy array (which rasterio.plot.show
is a wrapper of).
= plt.subplots()
fig, ax = plt.imshow(nz_elev.read(1), cmap='BrBG')
i ='BrBG', ax=ax);
rasterio.plot.show(nz_elev, cmap=ax); fig.colorbar(i, ax
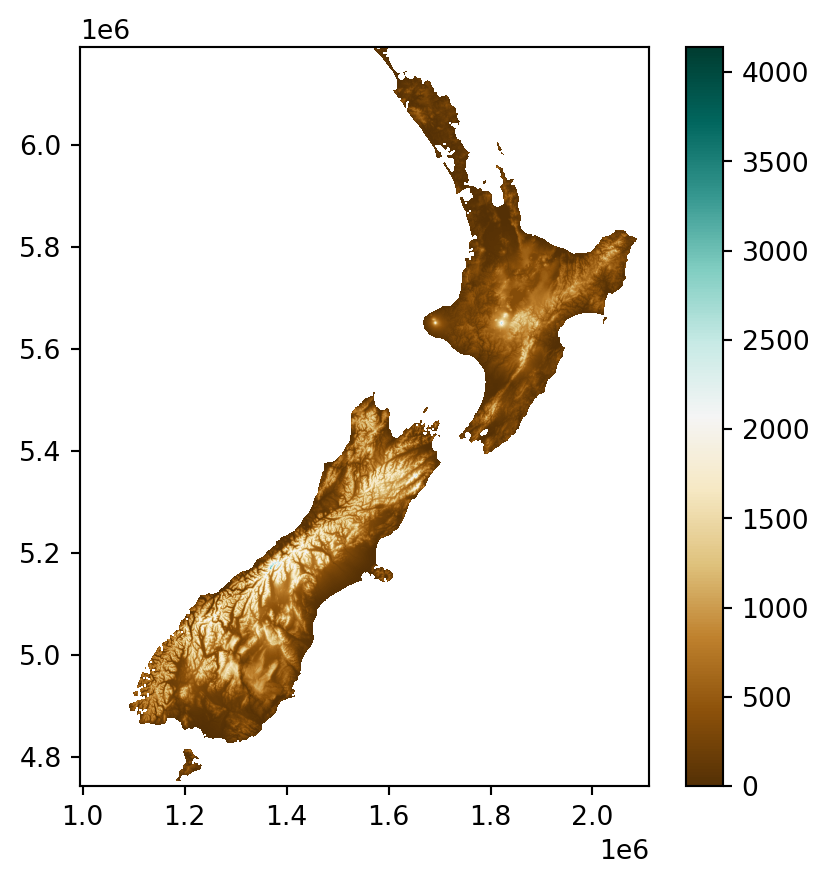
rasterio.plot.show
8.2.4 Labels
Labels are often useful to annotate maps and identify the location of specific features. GIS software, as opposed to matplotlib, has specialized algorithms for label placement, e.g., to avoid overlaps between adjacent labels. Furthermore, editing in graphical editing software is sometimes used for fine tuning of label placement. Nevertheless, simple labels added within the Python environment can be a good starting point, both for interactive exploration and sharing analysis results.
To demonstrate it, suppose that we have a layer nz1
of regions comprising the New Zealand southern Island.
= nz[nz['Island'] == 'South'] nz1
To add a label in matplotlib, we use the .annotate
method where the important arguments are the label string and the placement (a tuple
of the form (x,y)
). When labeling vector layers, we typically want to add numerous labels, based on (one or more) attribute of each feature. To do that, we can run a for
loop, or use the .apply
method, to pass the label text and the coordinates of each feature to .annotate
. In the following example, we use the .apply
method the pass the region name ('Name'
attribute) and the geometry centroid coordinates, for each region, to .annotate
. We are also using ha
, short for horizontalalignment
, with 'center'
(other options are 'right'
and 'left'
, see Text properties and layout reference for matplotlib) (Figure 8.11).
= plt.subplots()
fig, ax =ax, color='lightgrey', edgecolor='grey')
nz1.plot(axapply(
nz1.lambda x: ax.annotate(
=x['Name'],
text=x.geometry.centroid.coords[0],
xy='center'
ha
), =1
axis; )
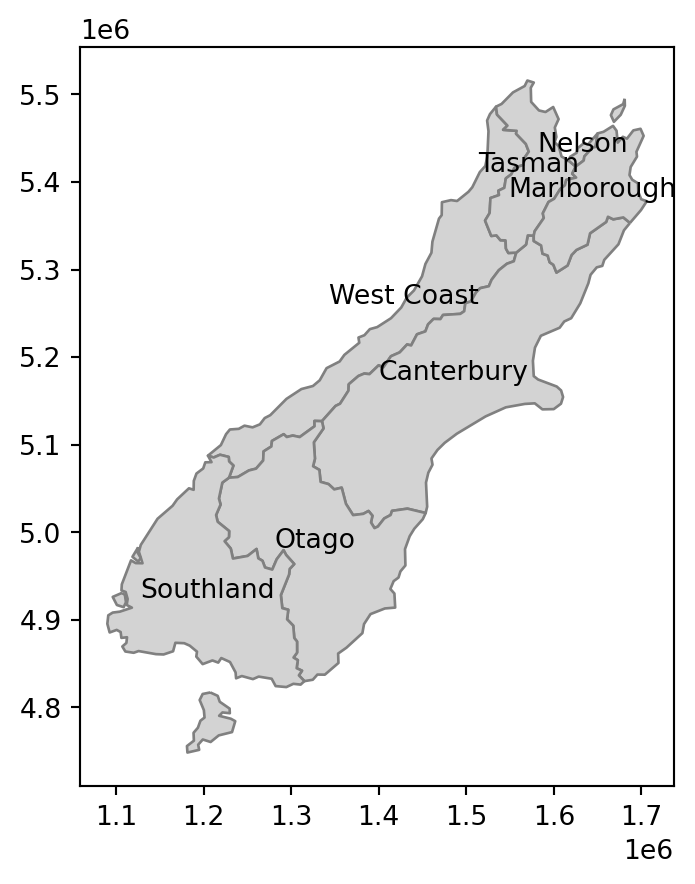
As another example, let’s create a map of all regions of New Zealand, with labels for the island names. First, we will calculate the island centroids, which will be the label placement positions.
= nz[['Island', 'geometry']].dissolve(by='Island').reset_index()
ctr 'geometry'] = ctr.centroid
ctr[ ctr
Island | geometry | |
---|---|---|
0 | North | POINT (18... |
1 | South | POINT (14... |
Then, we again use .apply
, combined with .annotate
, to add the text labels. The main difference compared to the previous example (Figure 8.11) is that we are directly passing the geometry coordinates (.geometry.coords[0]
), since the geometries are points rather than polygons. We are also using the weight='bold'
argument to use bold font (see Text properties and layout reference for matplotlib for list of other options) (Figure 8.12).
= plt.subplots()
fig, ax =ax, color='none', edgecolor='lightgrey')
nz.plot(axapply(
ctr.lambda x: ax.annotate(
=x['Island'],
text=x.geometry.coords[0],
xy='center',
ha='bold'
weight
), =1
axis; )
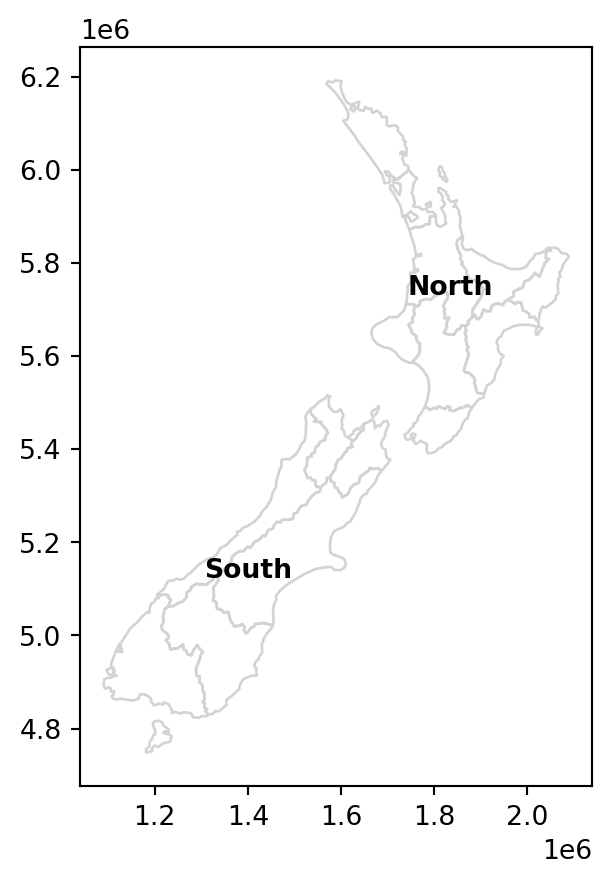
It should be noted that sometimes we wish to add text labels “manually”, one by one, rather than use a loop or .apply
. For example, we may want to add labels of specific locations not stored in a layer, or to have control over the specific properties of each label. To add text labels manually, we can run the .annotate
expressions one at a time, as shown in the code section below recreating the last result with the “manual” approach (Figure 8.13).
= plt.subplots()
fig, ax =ax, color='none', edgecolor='lightgrey')
nz.plot(ax'This is label 1', (1.8e6, 5.8e6), ha='center', weight='bold')
ax.annotate('This is label 2', (1.4e6, 5.2e6), ha='center', weight='bold'); ax.annotate(
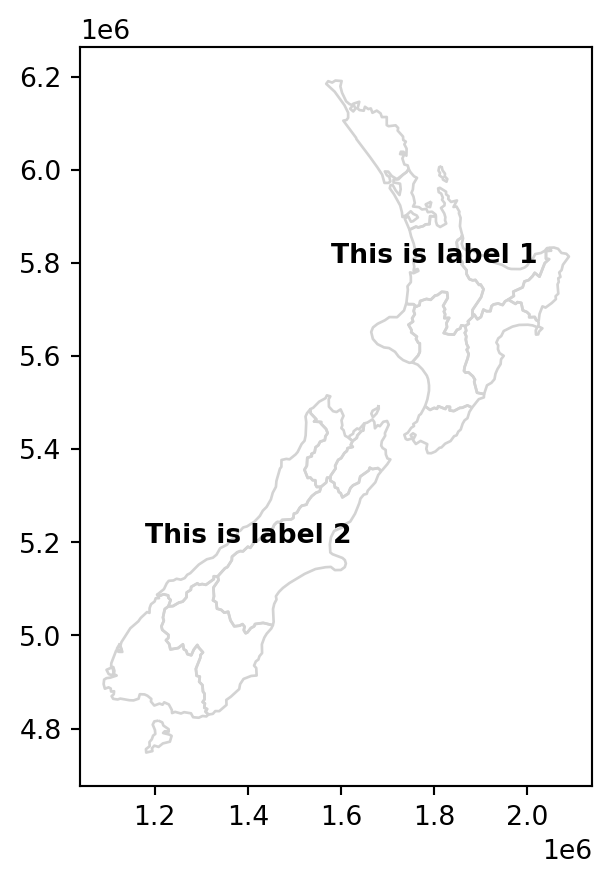
8.2.5 Layers
To display more than one layer in the same static map, we need to:
- Store the first plot in a variable (e.g.,
base
) - Pass it as the
ax
argument of any subsequent plot(s) (e.g.,ax=base
)
For example, here is how we can plot nz
and nz_height
together (Figure 8.14).
= nz.plot(color='none')
base =base, color='red'); nz_height.plot(ax
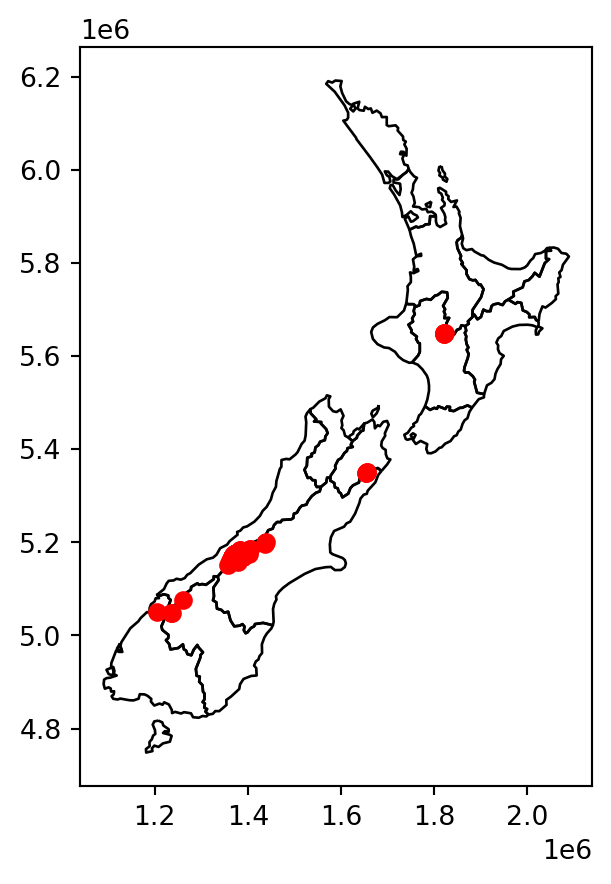
nz
(polygons) and nz_height
(points)
We can combine rasters and vector layers in the same plot as well, which we already used earlier in the book, for example when explaining masking and cropping (Figure 5.2). The technique is to initialize a plot with fig,ax=plt.subplots()
, then pass ax
to any of the separate plots, making them appear together.
For example, Figure 8.15 demonstrated plotting a raster with increasingly complicated additions:
- Panel (a) shows a raster (New Zealand elevation) and a vector layer (New Zealand administrative division)
- Panel (b) shows the raster with a buffer of 22.2 \(km\) around the dissolved administrative borders, representing New Zealand’s territorial waters (see Section 3.3.6)
- Panel (c) shows the raster with two vector layers: the territorial waters (in red) and elevation measurement points (in yellow)
# Raster + vector layer
= plt.subplots(figsize=(5, 5))
fig, ax =ax)
rasterio.plot.show(nz_elev, ax=ax, color='none', edgecolor='red');
nz.to_crs(nz_elev.crs).plot(ax# Raster + computed vector layer
= plt.subplots(figsize=(5, 5))
fig, ax =ax)
rasterio.plot.show(nz_elev, ax=nz.crs) \
gpd.GeoSeries(nz.unary_union, crs\
.to_crs(nz_elev.crs) buffer(22200) \
.\
.exterior =ax, color='red');
.plot(ax# Raster + two vector layers
= plt.subplots(figsize=(5, 5))
fig, ax =ax)
rasterio.plot.show(nz_elev, ax=nz.crs) \
gpd.GeoSeries(nz.unary_union, crs\
.to_crs(nz_elev.crs) buffer(22200) \
.\
.exterior =ax, color='red')
.plot(ax=ax, color='yellow'); nz_height.to_crs(nz_elev.crs).plot(ax
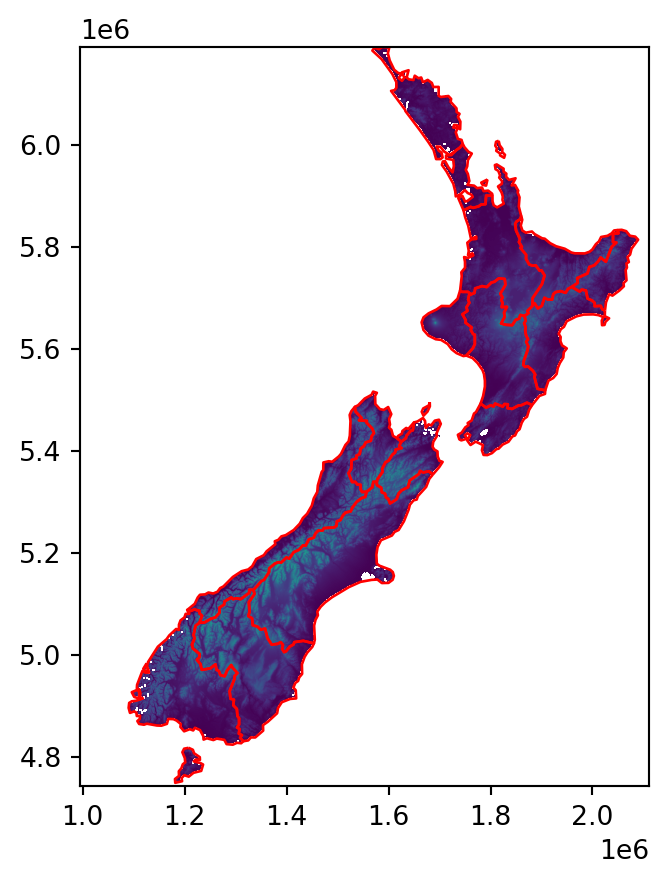
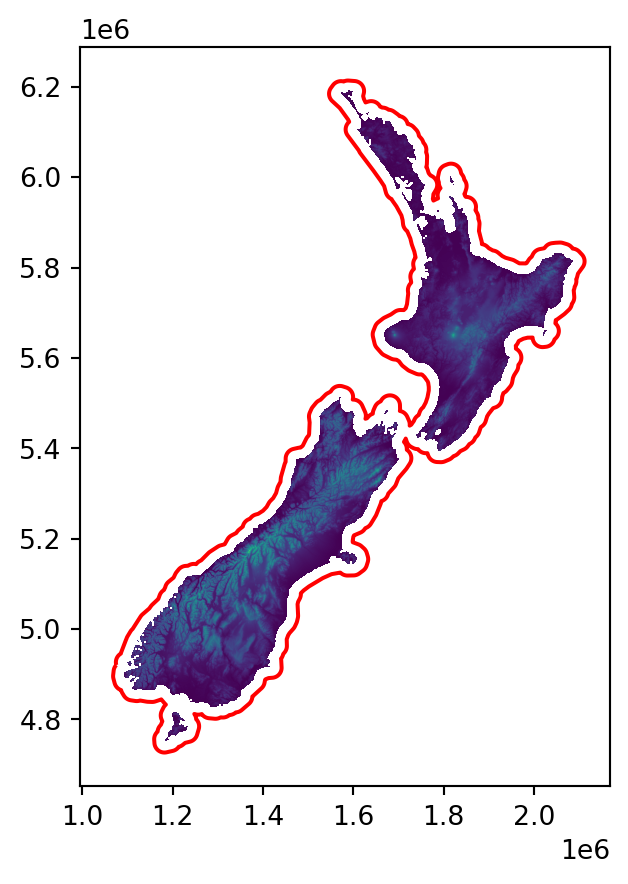
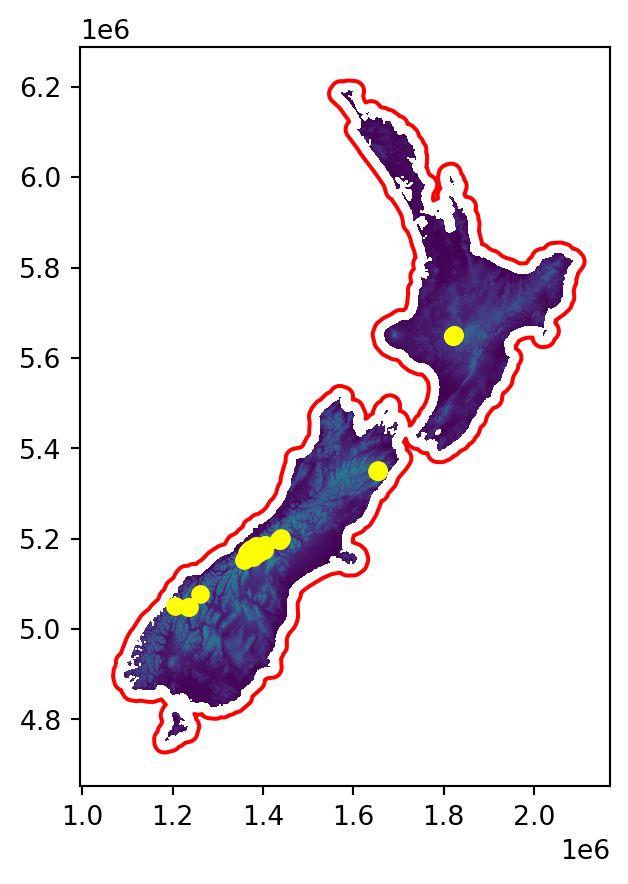
Note that the drawing order of layers is not necessarily according to the order of expressions, in the code, but according to layer type. For example, by default line layers are drawn on top of point layers. To override the default plotting order, we can use the zorder
argument of .plot
. Layers with higher zorder
values will be drawn on top. For example, the following would draw layer2
on top of layer1
(regaredless of their types).
= layer1.plot(zorder=1)
base =base, zorder=2); layer2.plot(ax
8.2.6 Basemaps
Basemaps, or background layers, are often useful to provide context to the displayed layers (which are in the “foreground”). Basemaps are ubiquitous in interactive maps (see Section 8.3). However, they are often useful in static maps too.
Basemaps can be added to geopandas static plots using the contextily package. A preliminary step is to convert our layers to EPSG:3857
(“Web Mercator”), to be in agreement with the basemaps, which are typically provided in this CRS. For example, let’s take the small "Nelson"
polygon from nz
, and reproject it to 3857
.
= nz[nz['Name'] == 'Nelson'].to_crs(epsg=3857) nzw
To add a basemap, we use the contextily.add_basemap
function, similarly to the way we added multiple layers (Section 8.2.5). The default basemap is “OpenStreetMap”. You can specify a different basemap using the source
parameter, with one of the values in cx.providers
(Figure 8.16).
# OpenStreetMap
= plt.subplots(figsize=(7, 7))
fig, ax = nzw.plot(color='none', ax=ax)
ax =cx.providers.OpenStreetMap.Mapnik);
cx.add_basemap(ax, source# CartoDB.Positron
= plt.subplots(figsize=(7, 7))
fig, ax = nzw.plot(color='none', ax=ax)
ax =cx.providers.CartoDB.Positron); cx.add_basemap(ax, source
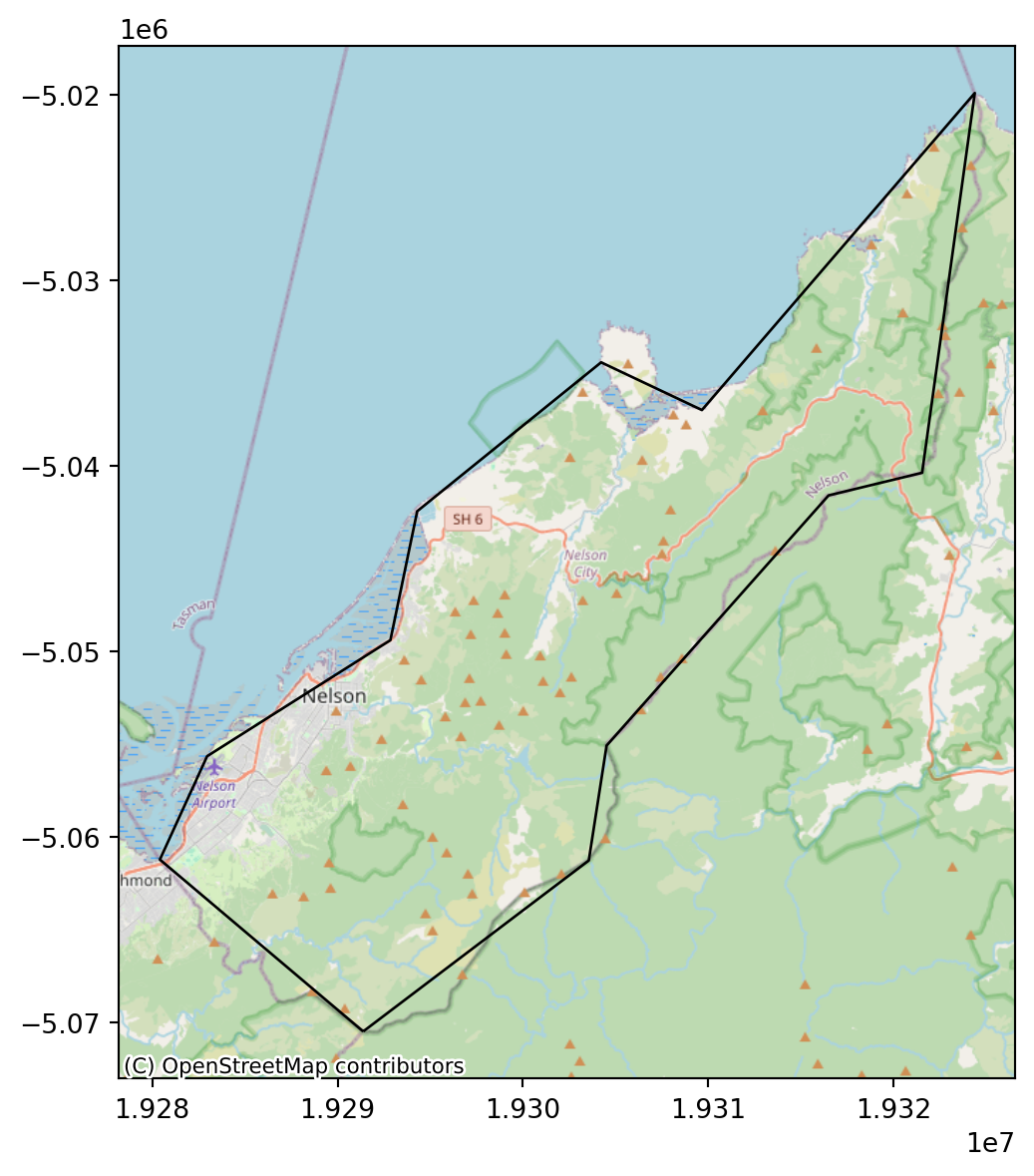
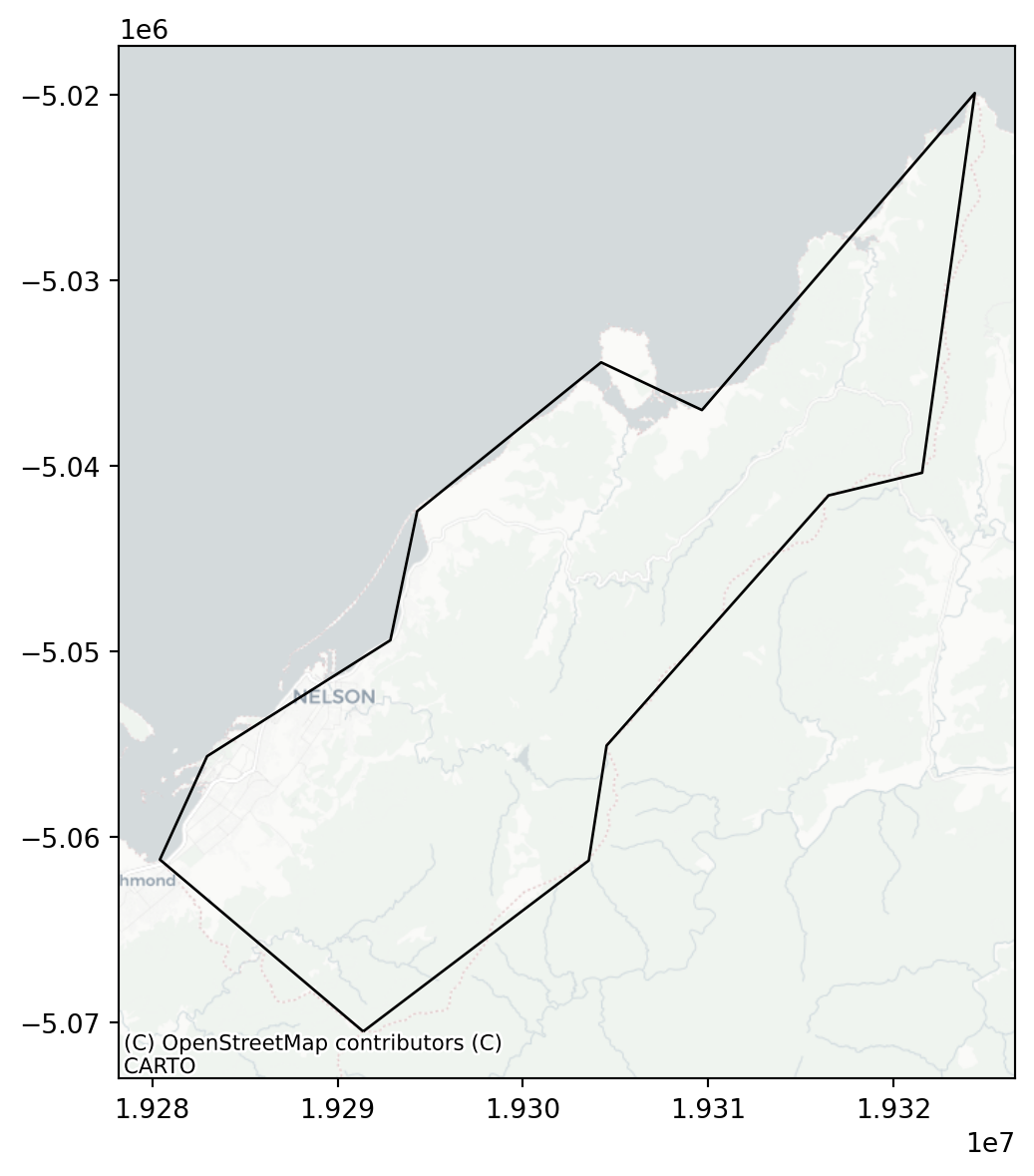
contextily
Check out the gallery for more possible basemaps. Custom basemaps (such as from your own raster tile server) can be also specified using a URL. Finally, you may read the Adding a background map to plots tutorial for more examples.
8.2.7 Faceted maps
Faceted maps are multiple maps displaying the same symbology for the same spatial layers, but with different data in each panel. The data displayed in the different panels typically refer to different properties, or time steps. For example, the nz
layer has several different properties for each polygon, stored as separate attributes:
vars = ['Land_area', 'Population', 'Median_income', 'Sex_ratio']
vars] nz[
Land_area | Population | Median_income | Sex_ratio | |
---|---|---|---|---|
0 | 12500.561149 | 175500.0 | 23400 | 0.942453 |
1 | 4941.572557 | 1657200.0 | 29600 | 0.944286 |
2 | 23900.036383 | 460100.0 | 27900 | 0.952050 |
... | ... | ... | ... | ... |
13 | 9615.976035 | 51100.0 | 25700 | 0.971898 |
14 | 422.195242 | 51400.0 | 27200 | 0.925967 |
15 | 10457.745485 | 46200.0 | 27900 | 0.957792 |
16 rows × 4 columns
We may want to plot them all in a faceted map, that is, four small maps of nz
with the different variables. To do that, we initialize the plot with the expected number of panels, such as ncols=len(vars)
if we wish to have one row and four columns, and then go over the variables in a for
loop, each time plotting vars[i]
into the ax[i]
panel (Figure 8.17).
= plt.subplots(ncols=len(vars), figsize=(9, 2))
fig, ax for i in range(len(vars)):
=ax[i], column=vars[i], legend=True)
nz.plot(axvars[i]) ax[i].set_title(
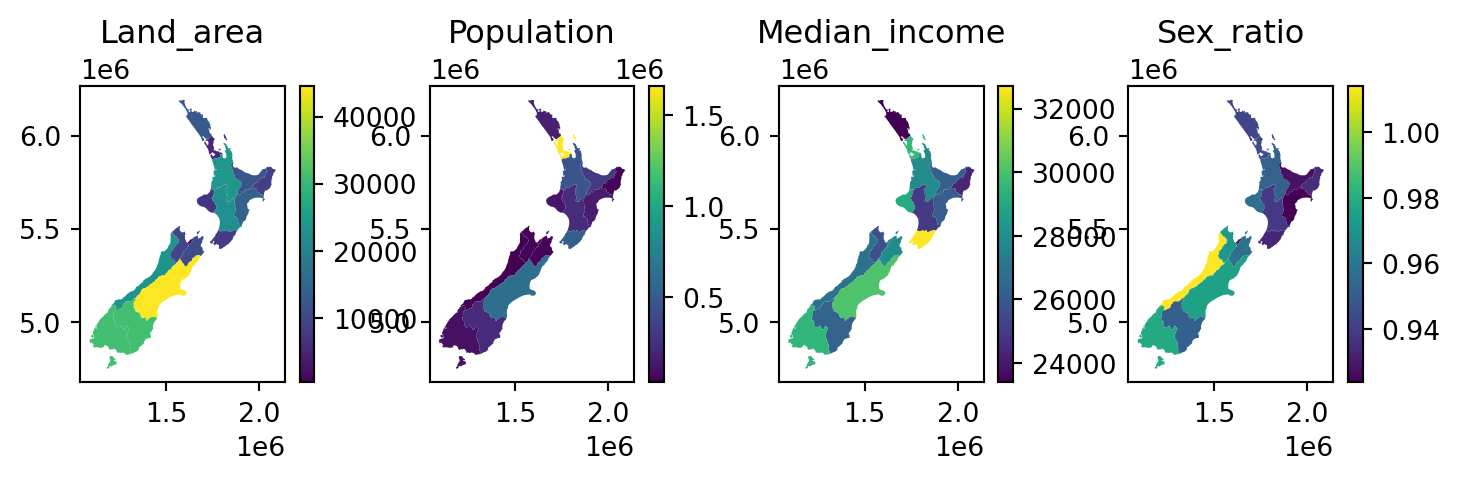
nz
In case we prefer a specific layout, rather than one row or one column, we can initialize the required number or rows and columns, as in plt.subplots(nrows,ncols)
, “flatten” ax
, so that the facets are still accessible using a single index ax[i]
(rather than the default ax[i][j]
), and plot into ax[i]
. For example, here is how we can reproduce the last plot, this time in a \(2 \times 2\) layout, instead of a \(1 \times 4\) layout (Figure 8.18). One more modification we are doing here is hiding the axis ticks and labels, to make the map less “crowded”, using ax[i].xaxis.set_visible(False)
(and same for .yaxis
).
= plt.subplots(ncols=2, nrows=int(len(vars)/2), figsize=(6, 6))
fig, ax = ax.flatten()
ax for i in range(len(vars)):
=ax[i], column=vars[i], legend=True)
nz.plot(axvars[i])
ax[i].set_title(False)
ax[i].xaxis.set_visible(False) ax[i].yaxis.set_visible(
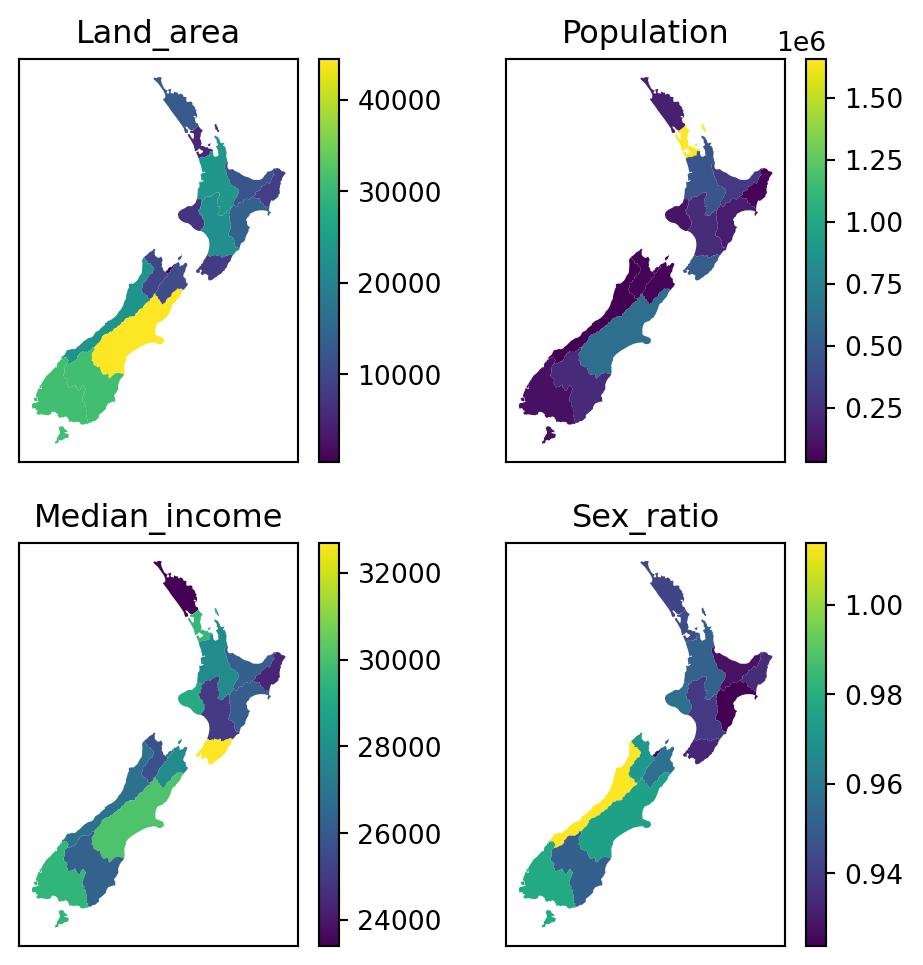
for
loop
It is also possible to “manually” specify the properties of each panel, and which row/column it goes in (e.g., Figure 2.2). This can be useful when the various panels have different components, or even completely different types of plots (e.g., Figure 5.4), making automation with a for
loop less applicable. For example, here is a plot similar to Figure 8.18, but specifying each panel using a separate expression instead of using a for
loop (Figure 8.19).
= plt.subplots(ncols=2, nrows=int(len(vars)/2), figsize=(6, 6))
fig, ax =ax[0][0], column=vars[0], legend=True)
nz.plot(ax0][0].set_title(vars[0])
ax[=ax[0][1], column=vars[1], legend=True)
nz.plot(ax0][1].set_title(vars[1])
ax[=ax[1][0], column=vars[2], legend=True)
nz.plot(ax1][0].set_title(vars[2])
ax[=ax[1][1], column=vars[3], legend=True)
nz.plot(ax1][1].set_title(vars[3]); ax[
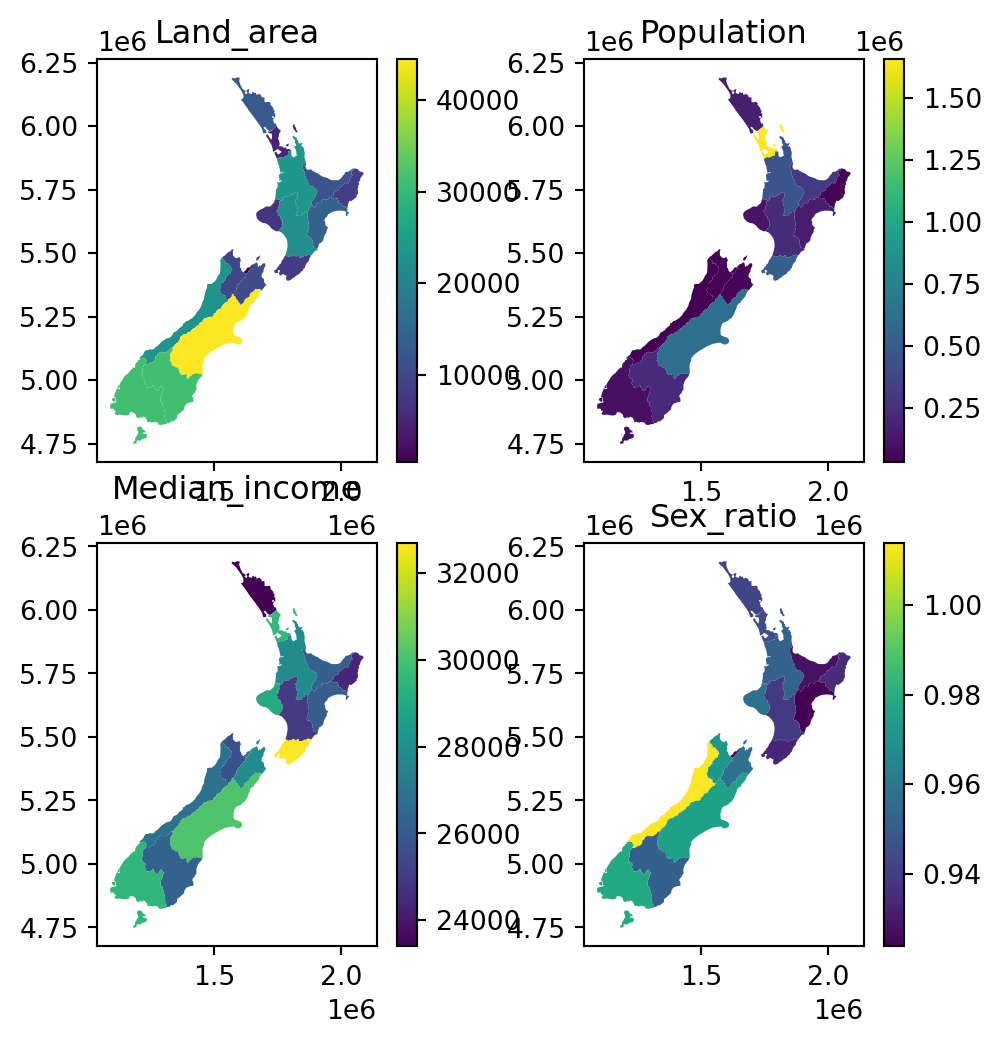
See the first code chunk in the next section for another example of manual panel contents specification.
8.2.8 Exporting
Static maps can be exported to a file using the matplotlib.pyplot.savefig
function. For example, the following code section recreates fig-two-layers, but this time the last expression saves the image to a JPG image named plot_geopandas.jpg
.
= nz.plot(color='none')
base =base, color='red');
nz_height.plot(ax'output/plot_geopandas.jpg') plt.savefig(
Figures with rasters can be exported exactly the same way. For example, the following code section (Section 8.2.5) creates an image of a raster and a vector layer, which is then exported to a file named plot_rasterio.jpg
.
= plt.subplots(figsize=(5, 5))
fig, ax =ax)
rasterio.plot.show(nz_elev, ax=ax, facecolor='none', edgecolor='r');
nz.to_crs(nz_elev.crs).plot(ax'output/plot_rasterio.jpg') plt.savefig(
Image file properties can be controlled through the plt.subplots
and plt.savefig
parameters. For example, the following code section exports the same raster plot to a file named plot_rasterio2.svg
, which has different dimensions (width = 5 \(in\), height = 7 \(in\)), a different format (SVG), and different resolution (300 \(DPI\)).
= plt.subplots(figsize=(5, 7))
fig, ax =ax)
rasterio.plot.show(nz_elev, ax=ax, facecolor='none', edgecolor='r');
nz.to_crs(nz_elev.crs).plot(ax'output/plot_rasterio2.svg', dpi=300) plt.savefig(
8.3 Interactive maps
While static maps can enliven geographic datasets, interactive maps can take them to a new level. Interactivity can take many forms, the most common and useful of which is the ability to pan around and zoom into any part of a geographic dataset overlaid on a ‘web map’ to show context. Less advanced interactivity levels include popups which appear when you click on different features, a kind of interactive label. More advanced levels of interactivity include the ability to tilt and rotate maps, and the provision of “dynamically linked” sub-plots which automatically update when the user pans and zooms (Pezanowski et al. 2018).
The most important type of interactivity, however, is the display of geographic data on interactive or ‘slippy’ web maps. Significant features of web maps are that (1) they eventually comprise static HTML files, easily shared and accessed by a wide audience, and (2) they can “grab” content (e.g., basemaps) or use services from other locations on the internet, that way providing detailed context without much requiring much effort from the person who created the map. The most popular approaches for web mapping, in Python and elsewhere, are based on the Leaflet JavaScript library (Dorman 2020). The folium Python package provides an extensive interface to create customized web maps based on Leaflet; it is recommended for highly-custimized maps. However, the geopandas wrapper .explore
, introduced in Section 1.2.2, can be used for a wide range of scenarios which are often sufficient. This is what we cover in this section.
8.3.1 Minimal example
An interactive map of a GeoSeries
or GeoDataFrame
can be created with .explore
(Section 1.2.2).
nz.explore()
.explore
8.3.2 Styling
The .explore
method has a color
parameter which affects both the fill and outline color. Other styling properties are specified using a dict
through style_kwds
(for general properties) and the marker_kwds
(point-layer specific properties), as follows.
The style_kwds
keys are mostly used to control the color and opacity of the outline and the fill:
stroke
—Whether to draw the outlinecolor
—Outline colorweight
—Outline width (in pixels)opacity
—Outline opacity (from0
to1
)fill
—Whether to draw fillfillColor
—Fill colorfillOpacity
—Fill opacity (from0
to1
)
For example, here is how we can set green fill color and 30% opaque black outline of nz
polygons in .explore
(Figure 8.21):
='green', style_kwds={'color':'black', 'opacity':0.3}) nz.explore(color
.explore
The dict
passed to marker_kwds
controls the way that points are displayed:
radius
—Curcle radius (in \(m\) forcircle
, see below) or in pixels (forcircle_marker
)fill
—Whether to draw fill (forcircle
orcircle_marker
)
Additionally, for points, we can set the marker_type
, to one of:
'marker'
—A PNG image of a marker'circle'
—A vector circle with radius specified in \(m\)'circle_marker'
—A vector circle with radius specified in pixels (the default)
For example, the following expression draws 'circe_marker
’ points with 20 pixel radius, green fill, and black outline (Figure 8.22).
nz_height.explore(='green',
color={'color':'black', 'opacity':0.5, 'fillOpacity':0.1},
style_kwds={'radius':20}
marker_kwds )
.explore
(using circle_marker
)
Figure 8.23 demonstrates the 'marker_type'
option. Note that the above-mentioned styling properties (other then opacity
) are not applicable when using marker_type='marker'
, because the markers are fixed PNG images.
='marker') nz_height.explore(marker_type
.explore
(using marker
)
8.3.3 Layers
To display multiple layers, one on top of another, with .explore
, we use the m
argument, which stands for the previous map (Figure 8.24).
= nz.explore()
m =m, color='red') nz_height.explore(m
.explore
One of the advantages of interactive maps is the ability to turn layers “on” and “off”. This capability is implemented in folium.LayerControl
from package folium, which the geopandas .explore
method is a wrapper of. For example, this is how we can add a layer control for the nz
and nz_height
layers (Figure 8.25). Note the name
properties, used to specify layer names in the control, and the collapsed
property, used to specify whether the control is fully visible at all times (False
), or on mouse hover (True
, the default).
= nz.explore(name='Polygons (adm. areas)')
m =m, color='red', name='Points (elevation)')
nz_height.explore(m=False).add_to(m)
folium.LayerControl(collapsed m
.explore
8.3.4 Symbology
Symbology can be specified in .explore
using similar arguments as in .plot
(Section 8.2.3). For example, Figure 8.26 is an interactive version of Figure 8.6 (a).
='Median_income', legend=True, cmap='Reds') nz.explore(column
.explore
Fixed styling (Section 8.3.4) can be combined with symbology settings. For example, polygon outline colors in Figure 8.26 are styled according to 'Median_income'
, however, this layer has overlapping outlines and their color is arbitrarily set according to the order of features (top-most features), which may be misleading and confusing. To specify fixed outline colors (e.g., black), we can use the color
and weight
properties of style_kwds
(Figure 8.27):
='Median_income', legend=True, cmap='Reds', style_kwds={'color':'black', 'weight': 0.5}) nz.explore(column
.explore
8.3.5 Basemaps
The basemap in .explore
can be specified using the tiles
argument. Several popular built-in basemaps can be specified using a string:
'OpenStreetMap'
'CartoDB positron'
'CartoDB dark_matter'
Other basemaps are available through the xyzservices package, which needs to be installed (see xyzservices.providers
for a list), or using a custom tile server URL. For example, the following expression displays the 'CartoDB positron'
tiles in an .explore
map (Figure 8.28).
='CartoDB positron') nz.explore(tiles
.explore
8.3.6 Exporting
An interactive map can be exported to an HTML file using the .save
method of the map
object. The HTML file can then be shared with other people, or published on a server and shared through a URL. A good free option for publishing a web map is through GitHub Pages.
For example, here is how we can export the map shown in Figure 8.25, to a file named map.html
.
= nz.explore(name='Polygons (adm. areas)')
m =m, color='red', name='Points (elevation)')
nz_height.explore(m=False).add_to(m)
folium.LayerControl(collapsed'output/map.html') m.save(